Implement a Virtual Robot
Table of content
Getting started
You need to fork and/or clone the repository, Pera-Swarm/java-robot into your environment, and setup by following the instructions on Java Robot.
You can create a new implementation of a Virtual Robot on the directory src/main/java/Robots
, with the following template. Rename MyTestRobot with the name of your Virtual Robot.
package Robots.samples;
import swarm.robot.VirtualRobot;
public class SampleRobot extends VirtualRobot {
public SampleRobot(int id, double x, double y, double heading) {
super(id, x, y, heading);
}
public void setup() {
System.out.println("My Test Robot");
super.setup();
}
public void loop() throws Exception {
super.loop();
if (state == robotState.RUN) {
System.out.println("Run");
} else if (state == robotState.WAIT) {
System.out.println("Waiting");
} else if (state == robotState.BEGIN) {
System.out.println("Begin");
}
}
@Override
public void communicationInterrupt(String msg) {
System.out.println("communicationInterrupt on " + id + " with msg:" + msg);
}
}
More sample Virtual Robot implementations are available in /src/main/java/Robots/samples/ of the repository
Virtual Robot State Machine
The virtual robot will change the state of it according to the following Finite State Machine.
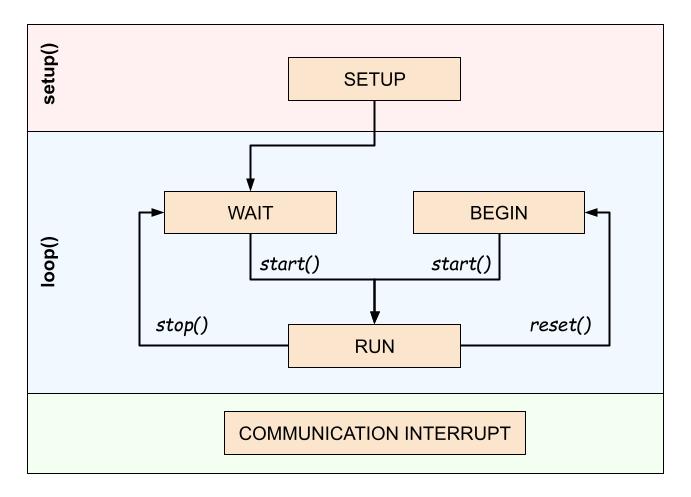
Default state changes
- setup(): This is the
public void setup()
method, which will be called once at the beginning of the robot initiation. - loop(): The rest of the states can be programmed within the
public void loop()
method.- START signal will change the state into the
robotState.RUN
- STOP signal will change the state into the
robotState.WAIT
- RESET signal will change the state into the
robotState.BEGIN
- START signal will change the state into the
Followings are the functions that will do the state changes. For any robot (either Physical or Virtual), the state changes can be done in the algorithm itself, or triggered via the Sandbox Tool.
- start() Can be triggered using a
START
signal/message - stop() Can be triggered using a
STOP
signal/message - reset() Can be triggered using a
RESET
signal/message
Communication Interrupt
Apart from the main 3 states, the Virtual Robots can receive interrupts. For now, there is only one interrupt type, known as communicationInterrupt
is there. It can be occurred at the end of the loop() iteration cycle.
This method can be used to handle the incoming messages as interrupts within public void communicationInterrupt(String msg)
.
public void communicationInterrupt(String msg) {
System.out.println("communicationInterrupt on " + id + " with msg:" + msg);
}
Override state changes
You can override the state changes by using the following Methods with @Override
within the Robot Class.
@Override
public void start() {
System.out.println("pattern start on " + id);
state = robotState.RUN;
}
@Override
public void stop() {
System.out.println("pattern stop on " + id);
state = robotState.WAIT;
}
@Override
public void reset() {
System.out.println("pattern reset on " + id);
state = robotState.BEGIN;
}
Run a Virtual Robot
- First, you need to start the Pera-Swarm Mixed Reality Simulator or select a
channel
of a running simulator. - Next, you need to configure the
App.java
file in the folder,/src/main/java/
, by creating one or more Virtual Robot Objects using the Class you implemented, by defining the start coordinates and heading directions of each Virtual Robot object. A detailed guide can be found on here. - Running instructions can be found on the Java Robot.
Additional Readings
- Autogenerated Documentation for the Java Robot can be found on https://pera-swarm.ce.pdn.ac.lk/robot-library-java/